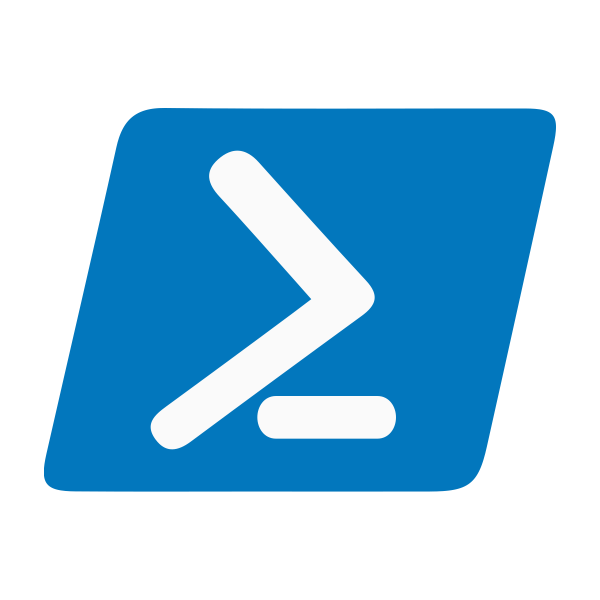
Automate RVTools Report Generation with PowerShell
One of my colleagues was manually running RVTools every day on five vCenter servers and exporting the results to Excel files on his client system. To streamline this process, I created a PowerShell script that automates the task. Here’s how you can set it up:
Step 1: Install RVTools
First, install RVTools on the system where you want to run the script.
Step 2: Test the RVTools Command
Open a command prompt (cmd) and run the following command to test the RVTools CLI:
"c:\program files (x86)\robware\rvtools\rvtools.exe" -s <vcenter ip address> -u <vcenter user> -p <vcenter password> -c ExportAll2xlsx -d <path to save the file> -f <file name>.xlsx
If the command works, proceed to the next step. If not, troubleshoot the credentials, network connectivity, or try installing another version of RVTools.
Step 3: Create the PowerShell Script
Open PowerShell ISE and copy the following script:
# Define variables
$vcenters = @("vcenter IP", "vcenter2 IP", "vcenter3 IP") # Add vCenter IP addresses
$RVToolsPath = "C:\Program Files (x86)\Robware\RVTools\RVTools.exe" # Path to RVTools.exe
$BaseReportPath = "C:\Users\fardad\Desktop\rvreport" # Destination folder
$LogFile = "$BaseReportPath\script_log.txt" # Log file
# Function to log messages
function Log-Message {
param (
[string]$message
)
$timestamp = Get-Date -Format "yyyy-MM-dd HH:mm:ss"
Add-Content -Path $LogFile -Value "$timestamp - $message"
}
# Function to create folder for the current month and year
function Get-ReportPath {
$currentDate = Get-Date
$folderName = $currentDate.ToString("yyyy-MM")
$reportPath = Join-Path -Path $BaseReportPath -ChildPath $folderName
if (-not (Test-Path -Path $reportPath)) {
New-Item -Path $reportPath -ItemType Directory | Out-Null
Log-Message "Created folder: $reportPath"
}
return $reportPath
}
# Function to delete reports older than one year
function Cleanup-OldReports {
$cutoffDate = (Get-Date).AddYears(-1)
$folders = Get-ChildItem -Path $BaseReportPath -Directory
foreach ($folder in $folders) {
if ($folder.LastWriteTime -lt $cutoffDate) {
Remove-Item -Path $folder.FullName -Recurse -Force
Log-Message "Deleted old folder: $($folder.FullName)"
}
}
}
# Generate new reports
$reportPath = Get-ReportPath
$currentDate = Get-Date -Format "yyyy-MM-dd"
foreach ($vcenter in $vcenters) {
$reportFile = "$reportPath\$currentDate-$vcenter.xlsx"
$Arguments = "-s $vcenter -u user -p pass -c ExportAll2xlsx -d $reportPath -f $reportFile"
Log-Message "Executing: $RVToolsPath $Arguments"
try {
$process = Start-Process -FilePath $RVToolsPath -ArgumentList $Arguments -Wait -NoNewWindow -PassThru
if ($process -ne $null) {
$process.WaitForExit()
$exitCode = $process.ExitCode
if ($exitCode -eq 0) {
Log-Message "Report generated for $vcenter"
} else {
Log-Message "RVTools exited with code $exitCode for $vcenter"
}
} else {
Log-Message "Failed to start RVTools process for $vcenter"
}
} catch {
Log-Message "Failed to generate report for $vcenter $($_.Exception.Message)"
}
}
This script creates a new folder each month and a subfolder every day, retaining the exported files for one year. Additionally, it generates a log each time the command runs.
Change the “vCenter IP addresses, user and password and any part of this script you wish and run a test.
If the test run successfuly you can save it as a .ps1 file.
Step 4: Schedule the Script
You can schedule this script to run daily using Windows Task Scheduler to ensure the reports are generated automatically.
Feel free to adjust the content as needed! If you have any questions or need further assistance, let me know.
Update
This script tested using rvtools 4.7
# This PowerShell script automates the generation of RVTools reports. To tailor the script to your infrastructure, update the specified variables accordingly. The script has been successfully tested with the latest RVTools version (4.7).
# Instructions:
# Install RVTools 4.7 on a Windows machine.
# Obtain the encrypted password.
# Update the variables in the script with your infrastructure details.
# Test the script to ensure it functions correctly.
# You can schedule this script to run daily using Windows Task Scheduler.
# Variables: ### Fields you should change ###
$rvtoolsPath = "C:\Program Files (x86)\Dell\RVTools\RVTools.exe" # The "RVTools.exe" path
$basePath = 'C:\path\to\save\reports' # Base Path of report files.
$vCenters = @("vCenter", "IP Address", "or FQDN") # vCenter Server FQDN or IP Addresses. Example: @("vcs1.domain.local", "192.168.1.201", ...)
$encryptedPassword = "_RVToolsLongLongLongEncryptedPassword" # Run command 'C:\Program Files (x86)\Dell\RVTools\RVToolsPasswordEncryption.ps1' in Powershell to get the encrypted password
$user = "vsphere.local\user" # vCenter Server User
$date = Get-Date
$monthPath = ($date).ToString("MM-yyyy")
$dayPath = ($date).ToString("dd-MM")
$fullMonthPath = Join-Path -Path $basePath -ChildPath $monthPath
$fullDayPath = Join-Path -Path $fullMonthPath -ChildPath $dayPath
$logFile = Join-Path -Path $basePath -ChildPath "Log.txt"
# Log Messages
Function Log-Message {
param (
[string]$message
)
$timeStamp = ($date).ToString("yyyy-MM-dd ; HH:mm:ss")
Add-Content -Path $logFile -Value "$timeStamp - $message"
}
# Create Month Directory:
if (-not (Test-Path -Path $fullMonthPath)){
New-Item -Path $basePath -Name $monthPath -ItemType Directory | Out-Null
}
# Create Day Directory:
if (-not (Test-Path -Path $fullDayPath)){
New-Item -Path $fullMonthPath -Name $dayPath -ItemType Directory | Out-Null
}
# Command Execution:
foreach ($vCenter in $vCenters){
$fileName = "$vCenter.xlsx"
$arguments = "-u $user -p $encryptedPassword -s $vCenter -c ExportAll2xlsx -d $fullDayPath -f $fileName"
$process = Start-Process -FilePath $rvtoolsPath -ArgumentList $arguments -Wait -NoNewWindow -PassThru
Log-Message "Running process with Id $($process.Id) for $vCenter"
if ($process -ne $null){
($process).WaitForExit()
$exitCode = ($process).ExitCode
if ($exitCode -eq 0){
Log-Message "Report generated for $vCenter"
} else {
Log-Message "Process exited with code $exitCode for $vCenter"
}
} else {
Log-Message "Failed to start RVTools process for $vCenter"
}
}